【第2回】Perlで画像処理 -エッジ検出-
現在のピクセルと周りのピクセルの色を調べて
フィルタリングしてやるとエッジを検出することができる。
■実行結果
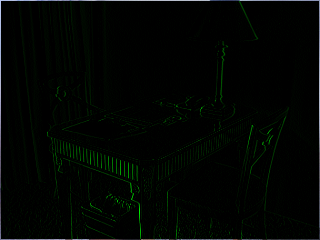
補正値は色々試してみたい気もする。
フィルタリングしてやるとエッジを検出することができる。
use strict;
use warnings;
use Imager;
use Image::Size;
my @filter = (
0, # upper_right
0, # top
0, # upper_right
0, # left
-1, # center
1, # right
0, # bottom_left
0, # bottom
0, # bottom_right
);
my $img = Imager->new;
my $set_img = Imager->new;
$img->read(file => 'test.png') or die $img->errstr;
$set_img->read(file => 'test.png') or die $img->errstr;
my ($max_width, $max_heigth) = imgsize('test.png');
for (my $x = 1; $x < $max_width - 1; $x++) {
for (my $y = 1; $y < $max_heigth - 1; $y++) {
my $upper_left_color = $img->getpixel(x => $x - 1, y => $y - 1);
my $top_color = $img->getpixel(x => $x, y => $y - 1);
my $upper_right_color = $img->getpixel(x => $x + 1, y => $y - 1);
my $left_color = $img->getpixel(x => $x - 1, y => $y);
my $center_color = $img->getpixel(x => $x, y => $y);
my $right_color = $img->getpixel(x => $x + 1, y => $y);
my $bottom_left_color = $img->getpixel(x => $x - 1, y => $y + 1);
my $bottom_color = $img->getpixel(x => $x, y => $y + 1);
my $bottom_right_color = $img->getpixel(x => $x + 1, y => $y + 1);
my @colors;
push (
@colors,
$upper_left_color,
$top_color,
$upper_right_color,
$left_color,
$center_color,
$right_color,
$bottom_left_color,
$bottom_color,
$bottom_right_color
);
my $red = 0;
my $green = 0;
my $blue = 0;
for (my $i = 0; $i < @colors; $i++) {
my ($tmp_red, $tmp_green, $tmp_blue, $alpha) =
$colors[$i]->rgba();
$red += $tmp_red * $filter[$i];
$green += $tmp_green * $filter[$i];
$blue += $tmp_blue * $filter[$i];
}
#$red = 255 if $red > 255;
#$red = 0 if $red < 0;
$red = 0;
$green = 255 if $green > 255;
$green = 0 if $green < 0;
#$green = 0;
#$blue = 255 if $blue > 255;
#$blue = 0 if $blue < 0;
$blue = 0;
my $after_color = Imager::Color->new($red, $green, $blue);
$set_img->setpixel(x => $x, y => $y, color => $after_color);
}
}
$set_img->write( file => 'save_test.png') or die $img->errstr;
■実行結果
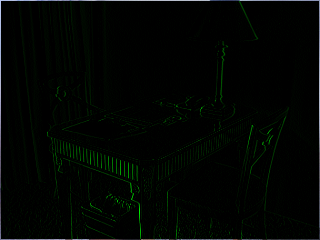
補正値は色々試してみたい気もする。